In RPG Developer Bakin, it's possible to do variable calculations, yes. However, it gets increasingly difficult to manage if you're trying to do complex calculations.
The aim of this guide is to show how more complex variable calculations can be done using C#.
Prerequisites
Ideally, you'll have at least a basic understanding of C# and how it works. It's fine if not as most of what I'm about to show you can be Googled anyway.
The Scenario
So, for our example, we've got a project where different "scores" are stored in variables.
To the end of our game, we want to calculate a "final score".
Our "scores" that we manage in Bakin are labelled as follows:
GameDesign
GameInnovation
EasterTheme
GameBugs
Our "final score" is labelled as "CalculatedScore" in Bakin.
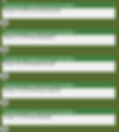
We want our final score to be the average of "GameDesign", "GameInnovation" and "EasterTheme", minus the value of "GameBugs".
Getting Prepped
So, before we get started, let's create a switch. It can be called whatever you'd like. I've named mine "CalculateScore". We'll use this to trigger our script once it's created.
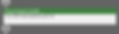
Next, we need to create a new custom common event in and attach an empty C# script to it called "CalculateScore.cs".
Note: We don't need to add anything else to this common event.

Edit the Script
Now that we're prepped, open your CalculateScore.cs file in your preferred code editor.
To start, remove the default methods in the script temporarily. Your file should look similar to this.

Next, we need to add the SystemData class and the name of our "CalculateScore" switch as variables.
Note: SystemData is what we use to access the variables and switches in Bakin.

Now we can add the Update method back into the script and create an "Initialise" method.
We'll then call the Initialise method in our Update method.
Note: The Initialise method is used to make sure the script has the basic data it needs to function.

Since the Update method is called every frame, we now want to check whether the "CalculateScore" switch is turned on. This way, we're only performing the calculations when we need to (which saves on computer resources).

Next, we'll get the current values of our "scores" and save them to variables in our script.

We're then going to perform the arithmetic operations we need to for our score.
Note: You can find the different arithmetic operations available in C# here.

Finally, we're going to round our score up to the nearest whole number and set the "CalculatedScore" variable in Bakin to the "calculatedScore" variable we have in our script.
We also turn off the "CalculateScore" switch in Bakin so we don't keep repeating the calculations.

Breakdown
This section breaks down what specific lines of code are doing in the image above:
Line 9 - Sets the _systemData variable. This is what we use to access Bakin's variables and switches.
Line 10 - Sets the name of "CalculateScore" variable in Bakin.
Lines 33 - 44 - Creates a method to make sure _systemData and mapScene (you don't need to know about mapScene) are not empty.
Line 14 - Runs the method we created on line 33.
Line 16 - Checks to see if the "CalcuateScore" switch in Bakin is true. This check is performed every once per frame in the game.
Lines 18 - 21 - Gets the different scores from Bakin and sets them to variables in our script.
Line 23 - First adds "gameDesign", "easterTheme" and "gameInnovation" together. Then, it divides the result by 3. Then, subtracts the value of "gameBugs" from the new result. The final result of that calculation is stored in the "finalScore" variable.
Line 25 - Rounds up the value of "finalScore" to the nearest whole number and stores the result in "calculatedScore".
Line 27 - Sets the "CalculatedScore" variable in Bakin to the value of "calculatedScore" from the script.
Line 29 - Turns off the "CalculateScore" switch to stop lines 18 - 29 from repeating unnecessarily.